Extending Portal Connector OAuth Registrations
Setup
The Portal Connector provides the ability to extend our default functionality of OAuth user registration. We use Ninject to provide the ability to switch the external auth handler based on the name of the registered Ninject binding, by default the name is “Default”. To create a custom binding, firstly you need to create a class and inherit from our base interface.
using System;
using Microsoft.Xrm.Sdk;
using pavliks.PortalConnector.UserManagement;
namespace SitefinityWebApp
{
public class CustomAuthUserHandler : ITpcExternalAuthUserHandler
{
public const string HandlerName = "CustomHandler";
public Entity CreatePortalUser(Guid sfUserId, ExternalProvider provider)
{
throw new NotImplementedException();
}
}
}
The next step is to register this custom handler with a Ninject binding, to do this add a InterfaceMappings.cs file to the root of your Sitefinity project and bind the interface to your custom class with the name.
using System;
using Ninject.Modules;
using pavliks.PortalConnector.UserManagement;
namespace SitefinityWebApp
{
public class InterfaceMappings : NinjectModule
{
public override void Load()
{
this.Bind().To().InSingletonScope().Named(CustomAuthUserHandler.HandlerName);
}
}
}
From here, all the code changes you need to make are done, simply compile and start the project. The last thing we need to do is tell The Portal Connector to use your custom handler instead of the default handler.
In the Sitefinity backend, navigate to Administration > Settings > Advanced
Select Authentication > SecurityTokenService > AuthenticationProviders
For each provider that you wish to bind this custom handler to, expand it, select parameters and click on “Create New”. Name this new parameter TpcExternalAuthProviderName and in the Value field put the name of the handler you defined in the steps above, in our case it is “CustomHandler”.
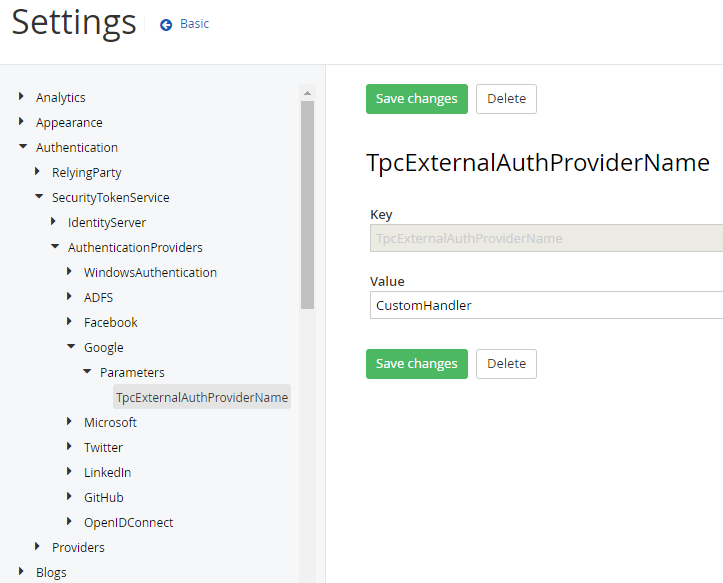
If you ever need to restore default functionality, simply delete this parameter or set the Value field to “Default”
The last step is to restart the AppPool for the settings to take affect.
And that’s it! In our example above, all OAuth user creation requests for the Google OAuth provider will instead be routed through your custom handler instead of The Portal Connector’s default handler.
Extending the Default Handler
If you don’t want to completely rewrite the user handler and only want to change a part of it, you can extend from our default handler instead of the interface. Below is an example of overriding our default class so you can extend the default functionality:
namespace SitefinityWebApp
{
public class CustomAuthUserHandler : DefaultTpcExternalAuthUserHandler
{
public const string HandlerName = "CustomHandler";
protected override Entity GetOrCreateUserContact(ICrmConnection connection, Guid sfUserId)
{
// Example: Change how the contact is retrieved instead of using emailaddress1 use some other field.
return base.GetOrCreateUserContact(connection, sfUserId);
}
public override Entity CreatePortalUser(Guid sfUserId, ExternalProvider provider)
{
Entity portalUser = base.CreatePortalUser(sfUserId, provider);
// Example: Create a CRM connection, modify the portal user and insert additional data.
return portalUser;
}
}
}
The base methods are all documented in the code and can be accessed using “base.” to see what they take in and what the methods do. The list of methods available for override are:
- SitefinityProfile GetSitefinityProfile(Guid sfUserId)
- Entity GetOrCreateUserContact(ICrmConnection connection, Guid sfUserId)
- Guid GetCurrentPortalId(ICrmConnection connection)
- Entity CreatePortalUser(Guid sfUserId, ExternalProvider provider)
- void UpdateProfile(Guid sfUserId, Entity contact)
Any questions regarding this API or how to extend this for your custom scenario, please don’t hesitate to contact us at support@crmportalconnector.com